Naming things¶
The debug layer might tell us possible leaks of various d3d11 related objects, the cool thing is, it can tell you exactly which object is leaking, via its name.
We can give our d3d11 objects a name.
Each D3D11 object (not the device itself) derives from ID3D11DeviceChild
and that interface
implements a method SetPrivateData
which we can use to assign a name to all those objects.
For that we will introduce a macro (hopefully the only one :))
template<UINT TDebugNameLength>
inline void SetDebugName(
_In_ ID3D11DeviceChild* deviceResource,
_In_z_ const char(&debugName)[TDebugNameLength])
{
deviceResource->SetPrivateData(WKPDID_D3DDebugObjectName, TDebugNameLength - 1, debugName);
}
And we use it like
SetDebugName(_deviceContext.Get(), "CTX_Main");
Unfortunately not every object is/implements ID3D11DeviceChild
so for the other things like
the dxgi factory or the device itself we have to use SetPrivateData
the ordinary way
constexpr char factoryName[] = "Factory1";
_factory->SetPrivateData(WKPDID_D3DDebugObjectName, sizeof(factoryName), factoryName);
constexpr char deviceName[] = "DEV_Main";
_device->SetPrivateData(WKPDID_D3DDebugObjectName, sizeof(deviceName), deviceName);
Now run the thing again and take a look at the summary report of the debug device in the output window
D3D11 WARNING: Live ID3D11Device at 0x000002214E8A1B10, Name: DEV_Main, Refcount: 3 [ STATE_CREATION WARNING #441: LIVE_DEVICE]
D3D11 WARNING: Live ID3D11Context at 0x000002214E8A5B50, Name: CTX_Main, Refcount: 0, IntRef: 1 [ STATE_CREATION WARNING #2097226: LIVE_CONTEXT]
Notice anything?
Exactly, they show names.
Naming things is also useful while debugging your application. In debuggers such as RenderDoc or Nvidia Nsight, the resource name will show up in the Resource Inspector, making it easier to find your resources and check its contents / configuration.
For example, if we name our Triangle vertex buffer using the follows
SetDebugName(_triangleVertices.Get(), "Triangle_Vertices");
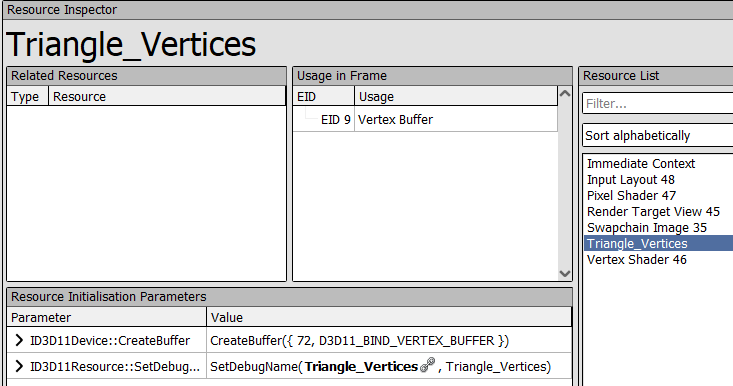
Similary, in Nsight's All Resources tab, we see